AJAX
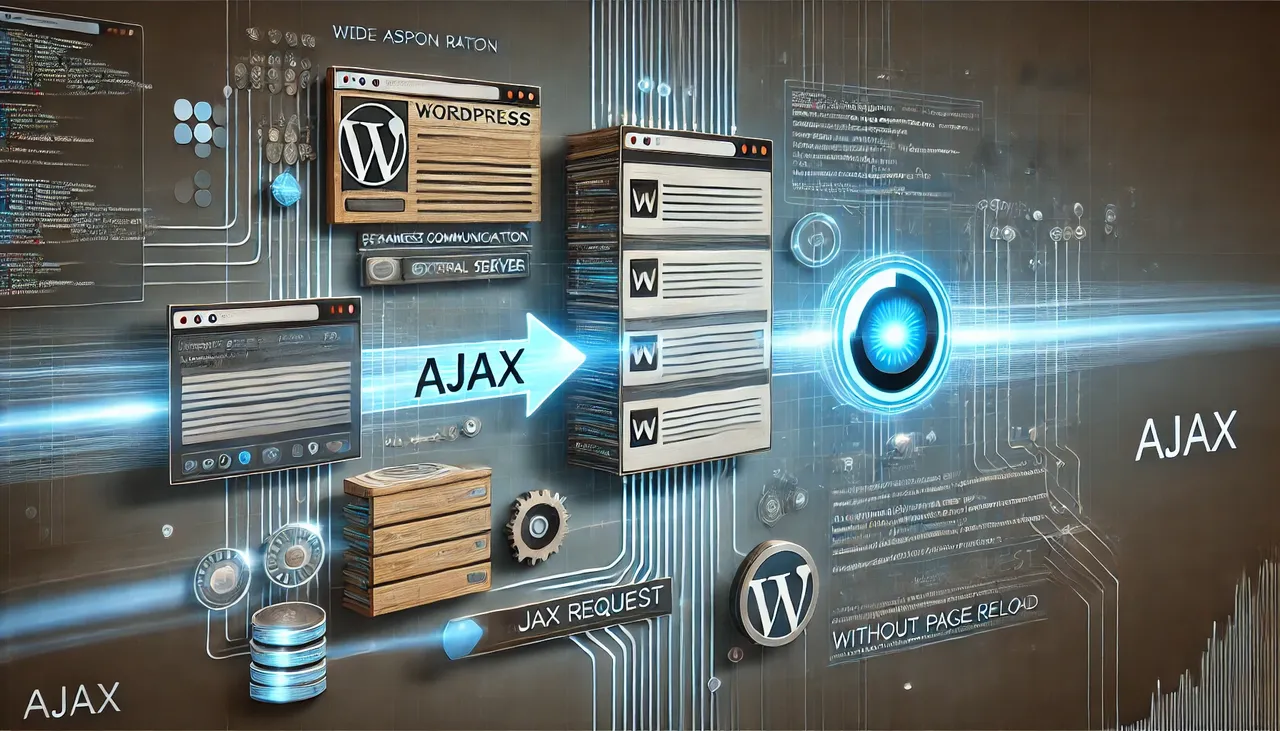
Understanding AJAX in WordPress – The use of AJAX in WordPress has become a fundamental technique for enhancing web page functionality.
By enabling dynamic updates without reloading the entire page, AJAX serves to optimize data transfers and provide instant changes.
This article aims to provide a comprehensive understanding of AJAX in the context of WordPress, covering both basic principles and advanced implementations.
Additionally, it will explore best practices for utilizing AJAX effectively.
Topics such as creating smart product searches, improving site search through plugins, implementing live AJAX searches, building AJAX forms, and incorporating infinite scroll will be discussed in detail.
Key Takeaways
Hide- AJAX in WordPress allows web pages to be updated without reloading the entire page.
- It is used in WordPress for actions like adding a new category or approving/deleting comments without page reload.
- AJAX makes WordPress websites faster by sending only necessary data back and forth, maximizing hosting bandwidth and improving efficiency.
- It enhances the user experience by reducing interruptions and allowing visitors to see changes instantly without page refresh.
What is AJAX? The Basics of AJAX in WordPress
The basics of AJAX in WordPress involve its definition as Asynchronous JavaScript and XML.
AJAX, which stands for Asynchronous JavaScript and XML, is a combination of web scripts and technologies that allows web pages to update specific sections without requiring a full page reload.
Its function in enabling web pages to be updated without reloading the entire page is particularly useful in common use cases for AJAX in WordPress, such as live search and infinite scroll.
In addition to its basic functionality, AJAX can also be utilized in various other functionalities within WordPress.
For example, it can be used for adding new categories or approving/deleting comments without page reload.
Furthermore, plugins can make use of AJAX to submit forms without reloading the page.
However, sometimes issues may arise with AJAX in WordPress, and troubleshooting these issues requires a thorough understanding of the underlying technologies and their implementation within the WordPress framework.
Advanced Implementations of AJAX in WordPress
Advanced implementations of AJAX in WordPress include the ability to create dynamic and interactive forms, incorporate live search functionality, and implement infinite scroll on a website.
These advanced form submissions leverage AJAX technology to submit form data without reloading the entire page, providing a seamless and efficient user experience.
With real-time updates, users can see changes instantly without the need for a page refresh, enhancing the responsiveness and interactivity of the website.
Live search functionality allows users to search for content on the website without navigating to a separate search page, saving time and providing a convenient search experience.
Additionally, implementing infinite scroll using AJAX enables users to continuously load content as they scroll, eliminating the need for pagination and providing a more immersive browsing experience.
These advanced implementations of AJAX in WordPress empower website owners to create powerful and user-friendly websites that optimize form submissions and provide real-time updates.
Best Practices for Using AJAX in WordPress
Optimizing the performance and functionality of a website involves implementing best practices for utilizing AJAX technology in the WordPress platform.
When using AJAX in WordPress, there are common pitfalls that developers should be aware of.
One common pitfall is relying too heavily on AJAX for functionality, which can lead to a slower website due to excessive server requests.
Another pitfall is not properly handling errors and validation, which can result in unexpected behavior and security vulnerabilities.
When implementing AJAX in WordPress, it is important to consider security considerations. AJAX requests should be properly authenticated and authorized to prevent unauthorized access to sensitive data or functionality.
Developers should also sanitize and validate user input to prevent vulnerabilities such as SQL injection or cross-site scripting attacks.
Additionally, implementing proper security measures such as using nonces and implementing rate limiting can further enhance the security of AJAX functionality in WordPress.
Overall, by being aware of common pitfalls and implementing appropriate security measures, developers can optimize the use of AJAX in WordPress while ensuring the security of the website.
Frequently Asked Questions (FAQs)
Can you use Ajax in WordPress?
Yes, you can use Ajax in WordPress to create dynamic and interactive user interfaces. WordPress comes with built-in Ajax handlers which you can use to implement Ajax functionality in both themes and plugins, allowing for seamless data exchange between the client and server without needing to reload the entire page.
What is Ajax in WordPress?
Ajax, which stands for Asynchronous JavaScript and XML, is a technique used to create fast and dynamic web pages. In the context of WordPress, Ajax is used to enable the interaction between the user’s browser and the server asynchronously, meaning that data can be exchanged, and parts of a page can be updated without requiring a full page reload. This results in a smoother and more responsive user experience. WordPress provides specific Ajax APIs to facilitate the implementation of Ajax functionality in themes and plugins.
How to write Ajax code in WordPress?
Writing Ajax code in WordPress involves several steps:
a. Enqueue JavaScript File: Firstly, enqueue a JavaScript file where you will write your Ajax functions using wp_enqueue_script()
function.
b. JavaScript Function: Write a JavaScript function to trigger the Ajax request. You will use the jQuery.ajax()
method to send data to the server and handle the response.
c. PHP Function: Create a PHP function to handle the Ajax request on the server-side. This function will process the data sent via Ajax and return a response.
d. Hooking PHP Function: Hook your PHP function to a WordPress Ajax action using add_action()
function.
e. Nonce Verification: For security, implement nonce verification to ensure that the Ajax request is coming from a legitimate source.
Here is a basic example:
<?php
// functions.php
function enqueue_custom_scripts() {
wp_enqueue_script('my-ajax-script', get_template_directory_uri() . '/js/ajax-script.js', array('jquery'), null, true);
wp_localize_script('my-ajax-script', 'my_ajax_object', array('ajax_url' => admin_url('admin-ajax.php')));
}
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
function handle_ajax_request() {
// Nonce verification
check_ajax_referer('my_ajax_nonce', 'nonce');
// Process the Ajax request
$response = array('success' => true, 'data' => 'Hello, World!');
wp_send_json($response);
}
add_action('wp_ajax_my_ajax_action', 'handle_ajax_request');
// ajax-script.js
jQuery(document).ready(function($) {
$.ajax({
url: my_ajax_object.ajax_url,
type: 'POST',
data: {
action: 'my_ajax_action',
nonce: my_ajax_object.nonce
},
success: function(response) {
console.log(response);
}
});
});
How to use Ajax in WordPress theme?
To use Ajax in a WordPress theme, follow the steps outlined in the answer to question 3. You would enqueue your JavaScript file, write a JavaScript function to handle the Ajax request, create a PHP function to process the request on the server-side, and hook this PHP function to a WordPress Ajax action.
This code would typically be placed in your theme’s functions.php
file and a custom JavaScript file within your theme’s directory. Remember to use nonce verification to secure your Ajax requests.