Array
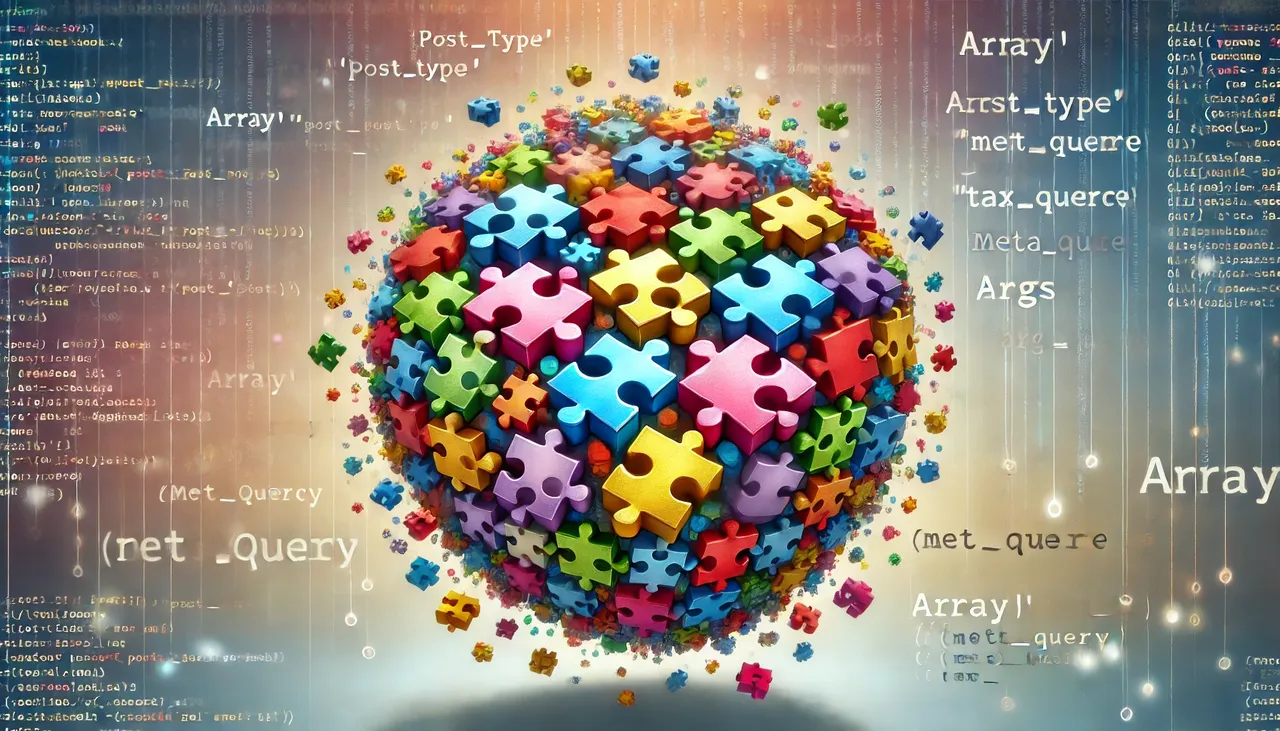
Understanding Array in PHP and WordPress – Arrays in WordPress functions are a crucial component of web development, offering a robust means of organizing and manipulating data efficiently.
With the ability to store multiple values under a single name, arrays provide a structured framework for managing data in WordPress themes, plugins, and core code.
By leveraging array functions such as count(), developers can streamline operations on multiple values, simplifying code complexity and enhancing readability.
This article explores the various types of arrays in WordPress and their practical applications, highlighting the benefits of incorporating arrays into WordPress functions.
Summary & Key Takeaways
Hide- Arrays in WordPress are special variables that can hold multiple values under a single name.
- They can be created using the array() function in PHP and can be indexed or associative.
- Multidimensional arrays contain more than one array and are commonly used in WordPress themes, plugins, and core code.
- Using arrays in WordPress helps in organizing and managing data efficiently, reducing code complexity, and improving readability.
What is an Array in WordPress Functions?
In WordPress, the term “array” doesn’t refer to a specific feature or function within the WordPress ecosystem, but rather to a fundamental programming concept that is used extensively in WordPress development, as it is in PHP programming more generally.
An array is a data structure that can hold more than one value at a time. It is a collection of elements identified by index or key.
In the context of WordPress, arrays are often used to handle various data, such as options, settings, and custom fields, among other things.
Here’s a brief explanation of different types of arrays and how they might be used in WordPress:
Indexed Arrays:
These have numeric indexes. For instance, when you’re querying multiple categories in WP_Query, you might use an indexed array to list the category IDs.
$args = array( 'category__in' => array( 4, 5 ) ); $query = new WP_Query( $args );
Associative Arrays:
These use named keys to identify values. WordPress functions and class methods often return data in the form of associative arrays.
$args = array( 'post_type' => 'post', 'posts_per_page' => 10 ); $query = new WP_Query( $args );
Multidimensional Arrays:
These are arrays that contain other arrays. WordPress options and settings are often stored as multidimensional arrays.
$args = array( 'tax_query' => array( array( 'taxonomy' => 'category', 'field' => 'slug', 'terms' => 'uncategorized' ) ) ); $query = new WP_Query( $args );
In WordPress development, you’ll often use arrays to pass arguments to functions, retrieve data from the database, and structure data in a way that’s easy to manipulate and access.
It’s a fundamental concept that you’ll encounter frequently when working with WordPress at a code level.
Benefits of Using Arrays in WordPress Functions
The use of arrays in WordPress functions offers benefits such as providing a structured way to store and access information, reducing code complexity, and improving readability.
In comparison to other data structures in WordPress functions, arrays provide a more efficient and organized approach to managing data.
Arrays allow developers to store multiple values under a single name, eliminating the need for separate variables and ensuring data integrity.
This is particularly useful in WordPress theme development, where arrays can be used to store and retrieve information such as theme options, widget settings, and menu configurations.
By using arrays, developers can easily loop through data, perform operations, and pass arrays as arguments to functions, enhancing the overall functionality and efficiency of WordPress themes.
Array Indexing and Accessing Values in WordPress Functions
Indexing in PHP starts at zero, allowing for specific elements to be accessed in an array. This feature is crucial when working with arrays in WordPress functions.
Arrays in WordPress not only provide a means of organizing and managing data efficiently but also offer powerful capabilities for accessing and manipulating values.
When dealing with multidimensional arrays in WordPress functions, array iteration methods become essential. These methods, such as foreach and array_map, allow for looping through the arrays and performing operations on each element.
Additionally, multidimensional arrays in WordPress functions enable the representation of more complex data structures, making it easier to work with hierarchical or nested data.
Overall, understanding array indexing and accessing values in WordPress functions is crucial for harnessing the full power of arrays in WordPress development.
Array Functions for Manipulating Data in WordPress Functions
Array functions for manipulating data provide a convenient way to perform operations on multiple values within the context of WordPress development.
These functions can be used to sort arrays, retrieve specific elements, merge multiple arrays, and more.
One common use case is sorting arrays in WordPress functions.
The sort()
function can be used to sort arrays in ascending order, while rsort()
can be used for descending order. Additionally, asort()
and arsort()
can be used to sort associative arrays based on their values.
However, when working with arrays in WordPress functions, it is important to be aware of common array errors and how to fix them.
Some common errors include accessing non-existent array elements, using incorrect keys, and improper array manipulation.
To fix these errors, it is recommended to use error handling techniques such as checking if an element exists before accessing it, validating keys before using them, and using appropriate array functions to manipulate data.