How to Allow SVG Uploads in WordPress Without Plugins
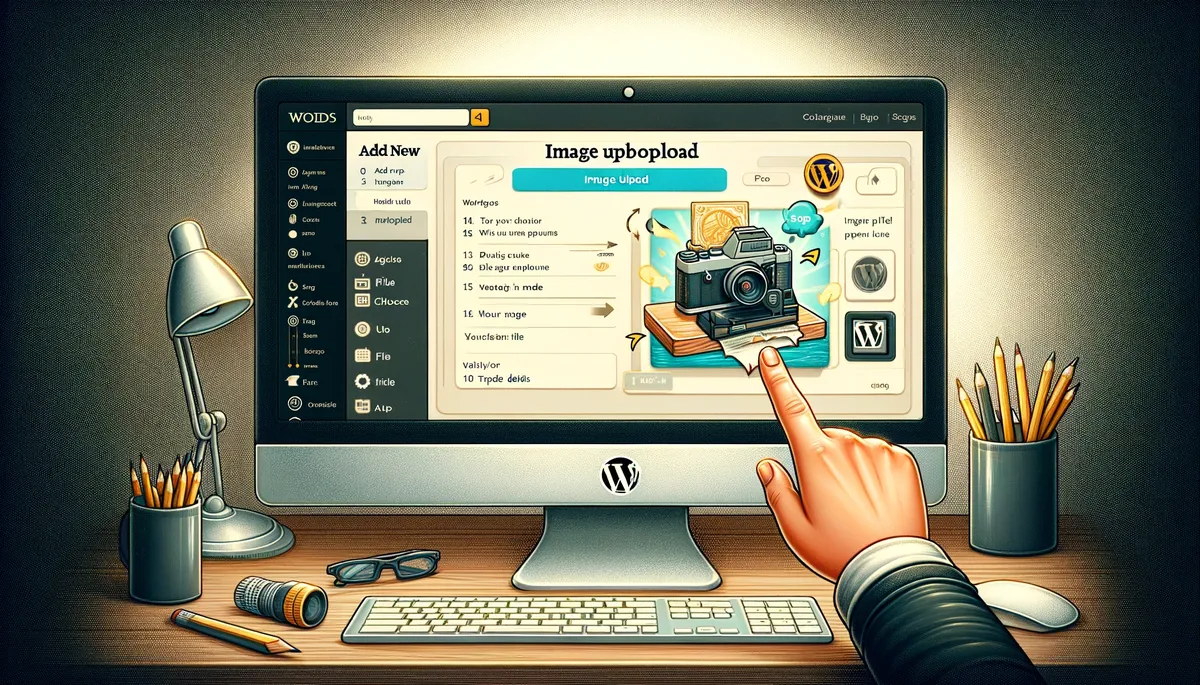
We detail allowing SVG uploads in WordPress while restricting access only to admins, avoiding plugins. SVG files pose security risks if enabled site-wide.
Our tutorial demonstrates safe, selective SVG access for trusted users through capability checking. Before implementation, consider if multimedia needs justify SVG risks given existing visual options.
Insert the Code Snippet into WordPress Child Theme or Code Snippet Plugin
Open your WordPress child theme’s functions.php file to start customizing your theme’s functionality. This can be done directly through the Theme Editor on your WordPress dashboard.
If you require more sophisticated edits, consider using an external code editor and upload your changes via FTP (File Transfer Protocol). To activate the new functionality, just add the given code snippet to the end of the functions.php
file.
add_filter(
'upload_mimes',
function ( $upload_mimes ) {
if ( current_user_can( 'manage_options' ) ) {
$upload_mimes['svg'] = 'image/svg+xml';
$upload_mimes['svgz'] = 'image/svg+xml';
}
return $upload_mimes;
}
);
Enhancing File Upload Capabilities
The provided code snippet enhances your site’s capabilities by adding a filter to upload_mimes
. This addition allows the uploading of SVG (image/svg+xml) and SVGZ (image/svg+xml) files.
It includes a safeguard that checks if the current user possesses the ‘manage_options’ capability, which is usually exclusive to administrators. This ensures that the ability to upload SVG files is restricted to administrator-level users.
Ensuring Security with SVG Files
While administrators are granted the ability to upload SVG files, it’s essential to validate and sanitize these files. This step is critical for maintaining the security of your website and preventing potential vulnerabilities.
Add the following function in your functions.php file:
// Validate and sanitize SVG files.
function explainwp_sanitize_svg_upload( $file ) {
$filetype = wp_check_filetype( $file, null );
if ( 'image/svg+xml' === $filetype['type'] ) {
$svg_content = file_get_contents( $file );
$svg_content = preg_replace( '/<script\b[^>]*>(.*?)<\/script>/is', '', $svg_content );
$svg_content = preg_replace( '/<!ENTITY(.*?)>/is', '', $svg_content );
$svg_content = preg_replace( '/<!DOCTYPE(.*?)>/is', '', $svg_content );
// Allow only specific SVG attributes.
$allowed_attributes = array( 'width', 'height', 'viewBox', 'xmlns' );
$svg_content = preg_replace( '/<svg(.*?)>/is', '<svg$1>', $svg_content );
$svg_content = preg_replace_callback(
'/<svg(.*?)>/is',
function ( $matches ) use ( $allowed_attributes ) {
$attributes = preg_replace( '/([a-zA-Z\-]+)="(.*?)"/is', '$1="$2"', $matches[1] );
$attributes = strip_tags( $attributes, '<' . implode( '><', $allowed_attributes ) . '>' );
return '<svg ' . $attributes . '>';
},
$svg_content
);
file_put_contents( $file, $svg_content );
}
return $file;
}
add_filter( 'wp_handle_upload_prefilter', 'explainwp_sanitize_svg_upload' );
Securing SVG File Uploads
The sanitize_svg_upload
function plays a crucial role in enhancing your website’s security. It meticulously sanitizes SVG files during the upload process.
This involves stripping out any potentially harmful code elements, like <script>
tags, and permitting only a select set of SVG attributes – width, height, viewBox, and xmlns – for heightened security.
After this sanitation process, the cleaned content is then saved back into the file, completing the upload process securely.
This function is seamlessly integrated into the wp_handle_upload_prefilter
, ensuring the sanitization occurs before WordPress processes the file.
This approach provides a robust way to allow SVG uploads in WordPress without the need for additional plugins. It’s a powerful feature that needs careful implementation, especially considering security aspects.
By adhering to these guidelines, you can enable SVG file uploads for administrators while ensuring each uploaded file undergoes thorough validation and sanitization, thereby reinforcing your website’s overall security.