PHP 8.0: The New PHP Version is Here!
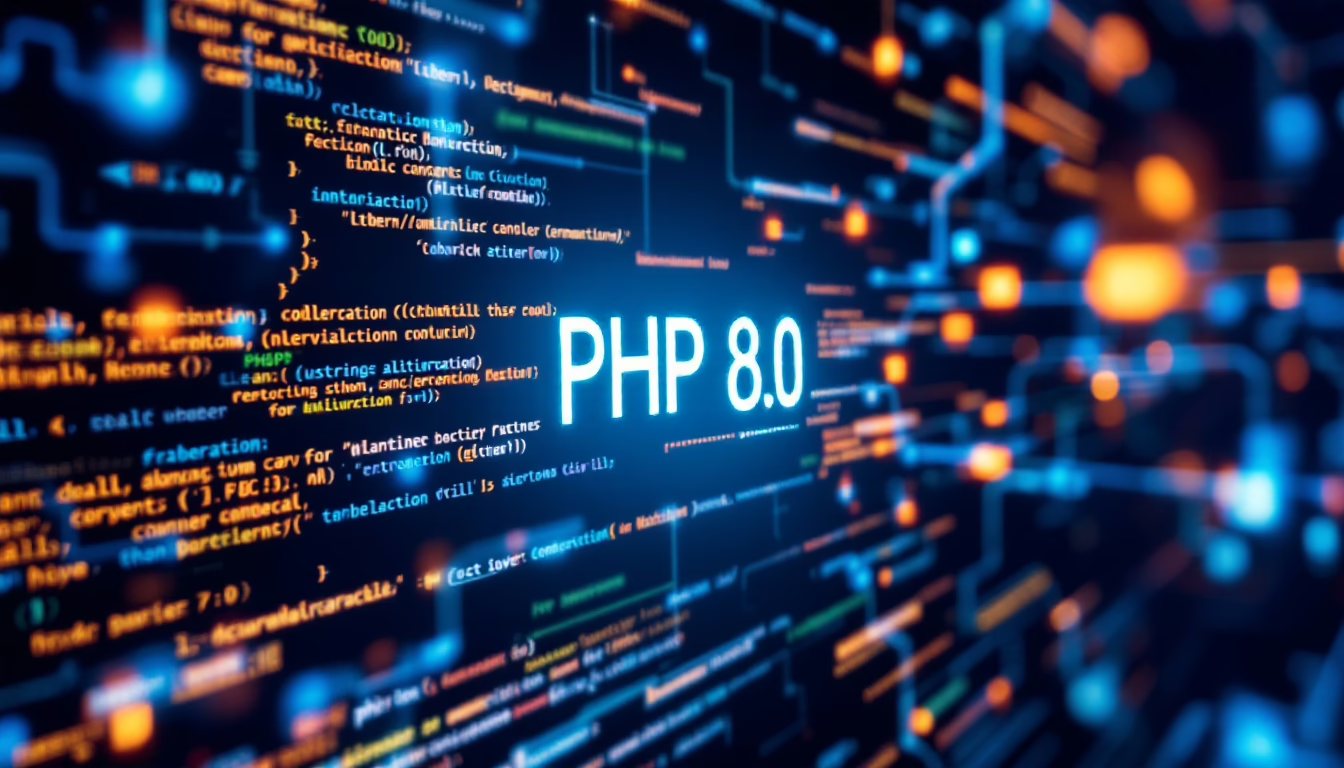
PHP 8.0: The New PHP Version is Here! – We’re excited to announce that PHP 8.0 is now available on the majority of hosting providers and servers capable of running WordPress.
This update includes new features and updates to help you get the most out of your web projects.
We encourage all users transitioning to the latest version to familiarize themselves with the new updates, which we will cover in this post, since this is a big update.
What Is PHP 8.0 and Why Do You Need It?
If you ask what is the latest PHP version, then as for now, PHP 8.0 is the latest PHP version right now.
According to Phoronix’s new benchmark tests, PHP 8.0 outperforms its predecessors by 10%. This data shows that PHP-based websites and applications have a bright future.
Even better, the test didn’t use the JIT compiler, a recent optimization function introduced with PHP 8.0. If it is allowed, developers can expect much faster results.
In addition, the latest version has new features that make coding much faster and cleaner by eliminating a lot of boilerplate and redundant code.
Since this is a version upgrade, if you upgrade to PHP 8.0 without making any modifications, your website will most likely encounter changes that may break it. We’ll guide you through all of the new features to help you plan for the migration.
What’s New in PHP 8.0?
Along with a slew of new features, the new update brings a slew of quality-of-life improvements. Let’s take a look at what’s fresh and different in PHP 8.0.
Just-in-Time (JIT) Compiler
One of the most exciting features of PHP 8.0 is the JIT compiler. This function aims to increase script execution efficiency by collaborating with opcache.
What is JIT?
The JIT, which stands for “just-in-time,” compiles opcode into machine code just before it is run for performance. To grasp what this means and how it works, we must first comprehend the PHP script execution steps, which are as follows:
- Dictionary/lexical analysis: Once the code has been processed by the Zend Engine, the PHP interpreter, the result is a list of machine-readable tokens.
- Parser: The interpreter breaks down the tokens into constituent parts in order to create an Abstract Syntax Tree (AST), which represents the code from a tree-like perspective.
- Compilation: When the interpreter converts the AST nodes into opcode, it does so so that the Zend VM (which executes in the memory of the PHP process) can know what operation to perform.
- Execution: In this case, the interpreter is delivering the opcode to the Zend VM, which compiles the opcode into machine code and then executes it.
The usage of server resources is significant in this process due to the number of PHP requests that will be repeated.
Because PHP 5.5 included the opcache extension, which stores the opcode from the compilation stage, this makes it possible to dynamically change the compiled script code without modifying the script source code.
Script execution is very fast on the server because when a new request comes in for the same script, the interpreter can immediately run the opcode from the opcache. Now that we’ve created an endless loop, there’s no need to go through the entire process again.
Even with PHP 7.4, which added a preloading feature several years later, having the opcache precompile scripts into opcode early on allows the PHP opcode to preload. Thus, the interpreter can deliver the instruction to be executed upon the first request to the script, after which the server sends it on to the next server in the chain.
The benefits listed above, although valuable, come with a number of disadvantages. One factor contributing to the long run time of the code is that at the end of the process, the Zend VM must convert the opcode into machine code before running it, which can take significant time and resources.
The JIT compiler is where all of that happens. The program will first compile the opcode to machine code during its first run, so that it will be ready for the next execution.
When there is a request for a JIT-compiled script, PHP will run it directly by the CPU rather than through the Zend VM, which results in better performance.
A single JIT compilation engine incorporates both JIT compilation and dynamic compilation, with the details as follows:
- Function. With this approach, you’ll be able to find and compile the entirety of a function, even though you won’t be able to identify the most frequently called functions.
- Tracing. This mode will only analyze and compile the most commonly used sections of the function, cutting down on the amount of time and memory it uses. In PHP 8.0, this is the default engine.
New Features in PHP 8.0
Furthermore, there are a multitude of exciting features other than just JIT. This section will provide an overview of the major new features and enhancements to PHP 8.0.
Union Types 2.0
In many cases, a function can use more than one type, but it was not possible to specify this in previous PHP versions unless you used DocComments to declare the types.
Here’s how it looks like:
class Number {
/**
* @var int|float $number
*/
private $number;
/**
* @param int|float $number
*/
public function setNumber($number) {
$this->number = $number;
}
/**
* @return int|float
*/
public function getNumber() {
return $this->number;
}
}
Previous PHP versions introduced two special union types: the Nullable type, which uses the ?Type syntax, and the Iterable type (for array and Traversable).
While a number of different language features were missing, the two most notable omissions were support for arbitrary union types, which was only available in PHP 8.0, and namespaces, which only became available in PHP 7.0. So, you can now simply define the types that the function can use, such as T1, T2, etc. and separate them using the T1|T2|… syntax, like so:
class Number {
private int|float $number;
public function setNumber(int|float $number): void {
$this->number = $number;
}
public function getNumber(): int|float {
return $this->number;
}
}
When you look at the example, you’ll notice that it doesn’t include the three variables @var, @param, and @return anymore, making the code much cleaner.
To return a value, you can use union types for properties, arguments, and return types. Though there are a few restrictions to keep in mind. You can check out the RFC for additional information.
Match Expressions
As a result, match expressions are very similar to switch statements, as their main purpose is to compare multiple values. In terms of performance, however, semantics are much more efficient and less prone to errors.
Let’s look at the following switch statement example from the RFC:
switch ($this->lexer->lookahead['type']) {
case Lexer::T_SELECT:
$statement = $this->SelectStatement();
break;
case Lexer::T_UPDATE:
$statement = $this->UpdateStatement();
break;
case Lexer::T_DELETE:
$statement = $this->DeleteStatement();
break;
default:
$this->syntaxError('SELECT, UPDATE or DELETE');
break;
}
When you have a match expression, the same statement will look considerably shorter:
$statement = match ($this->lexer->lookahead['type']) {
Lexer::T_SELECT => $this->SelectStatement(),
Lexer::T_UPDATE => $this->UpdateStatement(),
Lexer::T_DELETE => $this->DeleteStatement(),
default => $this->syntaxError('SELECT, UPDATE or DELETE'),
};
In the code block above, you can see that a match expression can return a value. Unlike a switch statement, where you must assign $result at the end, this assigns $result from the beginning.
Furthermore, there is no need to add a break after every arm because it has been implicitly implemented.
Furthermore, this feature will conduct a strict comparison instead of a loose one, thus conducting a strict equality comparison. In comparison to the switches found in programs, a loose comparison, as performed by the switch statements, can sometimes yield different results, resulting in bugs in your code. Now let’s take a look at the code that follows:
switch ('foo') {
case 0:
$result = "Oh no!\n";
break;
case 'foo':
$result = "This is what I expected\n";
break;
}
echo $result;
//> Oh no!
A match expression will present a more appropriate result:
echo match ('foo') {
0 => "Oh no!\n",
'foo' => "This is what I expected\n",
};
//> This is what I expected
It is important to remember that, in order for this feature to work properly, the arm must have a value that is equivalent to the condition. In addition, it is suggested that a default one be defined. If it isn’t handled, an UnhandledMatchError will be thrown.
Named Arguments
Prior to PHP 7, functions could only be invoked with a fixed number of arguments; therefore, passing additional arguments necessitated ensuring that the argument order positions in which the parameters were declared corresponded to the function call order, like so:
array_fill(0, 100, 50);
A complication with this is that you may not remember the order of parameters. Additionally, it can be difficult to understand what each of these variables refers to when you revisit the code.
With PHP 8.0, you have the choice of adding a name for the parameter to make it easier to refer to the parameter within a function using the parameter name rather than its number. Here’s what it looks like most of the time:
// Using positional arguments:
array_fill(0, 100, 50);
// Using named arguments:
array_fill(start_index: 0, num: 100, value: 50);
Additionally, through this feature, we are able to process things much more quickly because we will know the exact effects of each parameter.
Attributes
An attribute is a metadata function that is capable of describing what a particular section of code means. If you place an element in functions, classes, class constants, class properties, class methods, or function or method parameters, you can expand the element.
In terms of features, this one is similar to DocComments, though there are a few differences.
It is widely understood in the PHP development community that the fact that it is native to the PHP system makes it readable to tools like static analyzers and syntax highlighters. In the RFC, it is proposed that attributes be used as a more structured and syntax-based approach to metadata.
In this manner, it is possible to mark the individual attributes that are mentioned within the example text such as <<Example>> As shown, you can place them prior to their declaration statements, such as this:
<<ExampleAttribute>>
class Foo
{
<<ExampleAttribute>>
public const FOO = 'foo';
<<ExampleAttribute>> public $x;
<<ExampleAttribute>> public function foo(<<ExampleAttribute>> $bar) { } }
Furthermore, users can include a single or multiple associated values in the attribute, as demonstrated below:
<<WithoutArgument>>
<<SingleArgument(0)>>
<<FewArguments('Hello', 'World')>>
function foo() {}
To specify an attribute before or after a DocComment, go ahead and attach it like this:
<<ExampleAttribute>>
/** docblock */
<<AnotherExampleAttribute>>
function foo() {}
Additional PHP 8.0 Changes
The items listed below will not introduce backward incompatibility, but it is still recommended that you take note of them. These are some of the things to look for:
- Filter results from ReflectionClass::getConstants() and ReflectionClass::getReflectionConstants() by using the new filter parameter.
- There is a new argument called flags for ZipArchive::addEmptyDir(), ZipArchive::addFile() and ZipArchive::addFromString() methods.
- The ZipArchive::addGlob() and ZipArchive::addPattern() methods now accept flags, comp_method, comp_flags, env_method, and enc_password in the options array.
- Due to its essential role in PHP, the JSON extension is now enabled by default.
- The array_slice() function won’t have to find the starting position if the array doesn’t have gaps.
For more information regarding what’s new in PHP 8.0, you can read more on the official PHP.net page.
Combining WordPress with PHP 8.0
Widgets created in WordPress 5.6 and up will work with PHP 8.0. The developer team has removed many obsolete features in order to make this happen.
To put it another way, however, since many plugins and themes haven’t supported PHP 8.0 yet, they cannot make a full claim of compatibility.
Before making the move to PHP 8.0, ensure that your code is not likely to cause any problems when shifting to the new version. Otherwise, if you’re working with a professional web developer, approach the developer and inquire whether they could assist with the transition.
Also Read: How to Start a WordPress Blog: The Easiest Guide
As a plugin or theme developer, WordPress encourages making adjustments to your product’s code so that it is compatible with the new PHP version. In that way, plugin or theme users will be spared from having to deal with new software updates that will break their website if they make a change.
This article has laid out the major alterations that may be implemented in the lives of WordPress developers. We’d like to add that, while we believe strongly in the following statements, it is strongly recommended that you read the following WordPress announcement to learn which areas to focus on.
Although there are still a number of issues to be resolved, you may always lower your PHP version to PHP 7.4 if things get out of hand.
Wrapping Up
There are a few exciting changes and improvements for PHP developers in PHP 8.0. Website performance and code-writing experience are expected to see a boost because of constructors property promotion, which has recently become available in the JIT compiler.
Make sure to check out the RFCs and documentation, as well as any manuals that might apply, so that your projects will be compatible with the latest PHP version. Let’s go, good luck!